Getting Started
Get started with EveryLog in 2 minutes.
Step 1: Sign up
Sign up to EveryLog with your email.
Step 2: Get the API Key
After confirming your email, you'll be able to access EveryLog and obtain your personal API Key.
The API Key is needed to make calls from your channels and applications.
Step 3: Download the mobile app
Download the mobile app from Google or Apple App stores, then sign in to your EveryLog account.
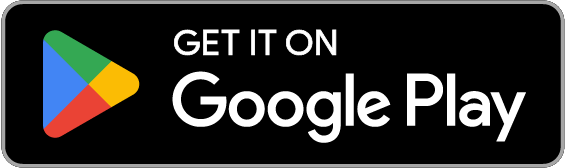
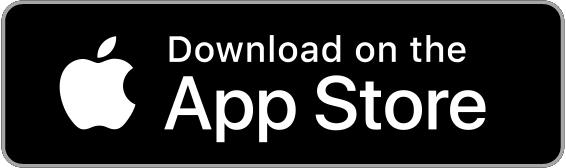
Step 4: Add a post in your code
Add a POST request to the EveryLog API in your code and you'll be all set!
Your app will receive its first notification.
The minimum set of required params is:
- projectId
- title
- summary
- body All others params are not mandatory
Sample code for post
Javascript
javascript example
import axios from 'axios';
const headers = { 'Authorization': `Bearer ${API_KEY}` }
const data = {
projectId: "project-name",
title: "New subscription",
summary: "A new user has subscribed to base plan",
body: "foo@bar.com just subscribed to plan XYZ",
tags: [
"new subscriber",
"welcome"
],
groups: [
"first-group",
"second-group"
],
roles: [
"admin",
"developers"
],
users: [
"user1@example.com",
"user2@example.com"
],
properties: {
key: "value",
key2: "value2"
},
externalChannels: [
"channel-code",
"other-channel-code"
],
icon: "🤪",
link: "https://www.example.com",
push: true
}
axios.post("https://api.everylog.io/api/v1/log-entries", data, { headers: headers });
Ruby
ruby example
require 'rest-client'
require 'json'
api_key = 'XYZ'
everylog_url = "https://api.everylog.io/api/v1/log-entries"
body = {
projectId: "project-name",
title: "New subscription",
summary: "A new user has subscribed to base plan",
body: "foo@bar.com just subscribed to plan XYZ",
tags: [
"new subscriber",
"welcome"
],
groups: [
"first-group",
"second-group"
],
roles: [
"admin",
"developers"
],
users: [
"user1@example.com",
"user2@example.com"
],
properties: {
key: "value",
key2: "value2"
},
externalChannels: [
"channel-code",
"other-channel-code"
],
icon: "🤪",
link: "https://www.example.com",
push: true
}
RestClient.post(everylog_url,
body.to_json,
{Authorization: "Bearer #{api_key}",
content_type: :json})
Python
python example
import requests
api_key = 'XYZ'
headers = {"Authorization": "Bearer %s" % api_key}
body = {
"projectId": "project-name",
"title": "New subscription",
"summary": "A new user has subscribed to base plan",
"body": "foo@bar.com just subscribed to plan XYZ",
"tags": [
"new subscriber",
"welcome"
],
"groups": [
"first-group",
"second-group"
],
"roles": [
"admin",
"developers"
],
"users": [
"user1@example.com",
"user2@example.com"
],
"properties": {
"key": "value",
"key2": "value2"
},
"externalChannels": [
"channel-code",
"other-channel-code"
],
"icon": "🤪",
"link": "https://www.example.com",
"push": False
}
r = requests.post(url="https://api.everylog.io/api/v1/log-entries", json=body, headers=headers)
Curl
curl example
curl --request POST \
--url https://api.everylog.io/api/v1/log-entries \
--header 'Authorization: Bearer XXX-YYY-ZZZ' \
--header 'Content-Type: application/json' \
--header 'charset:utf-8' \
--data '{
"projectId":"project-name",
"title":"New subscription",
"summary":"A new user has subscribed to base plan",
"body": "foo@bar.com just subscribed to plan XYZ",
"tags":[
"new subscriber",
"welcome"
],
"groups": [
"first-group",
"second-group"
],
"roles": [
"admin",
"developers"
],
"users": [
"user1@example.com",
"user2@example.com"
],
"externalChannels": [
"channel-code",
"other-channel-code"
],
"properties": {
"key": "value",
"key2": "value2"
},
"icon": "🤪",
"link": "https://www.example.com",
"push": true
}'
Supported HTML Tags
In the body parameter you can pass not only text but also a block of HTML code. The supported tags are:
Headings
<h1></h1>
<h2></h2>
<h3></h3>
<h4></h4>
<h5></h5>
<h6></h6>
Typography
<br/>
<p></p>
<b></b>
<i></i>
<u></u>
Link and images
<a href="..."></a>
<img src="..." />
Lists
<ul></ul>
<ol></ol>
<li></li>